Overflow problems may frustrate and confuse both experienced and rookie programmers. In the middle of your project, carefully drafting lines of code, you get an "overflow error." What does it mean? How did it happen? And most importantly, how do you correct it?
Overflow Errors:
Do not worry, programmer! This blog article will explain overflow faults, their origins, detection methods, and practical remedies to help you overcome these notorious issues. Grab your virtual magnifying glass, and let's explore overflow faults!
Overflow Error Common Causes
Data type overflow problems arise when a value exceeds its maximum storage limit. These flaws can cause program failures, unusual behavior, or inaccurate results. Preventing and fixing overflow issues requires understanding their causes.
Overflow problems sometimes result from arithmetic operations with values too big for their data types. Adding two big integers may cause an overflow error if the sum exceeds the data type's maximum value.
Overflow issues can also result from poor user input or external data management. If input validation is incorrect, users or external sources may submit values that exceed the application's restrictions, causing overflow problems.
Overflow issues can also result from programming errors like utilizing wrong variables or ignoring boundary conditions. Make sure that code logic and computations are within acceptable bounds.
Overflow faults can also result from memory allocation and resource management concerns. Overflowing memory buffers can cause program crashes if they are not adequately allocated or handled.
To avoid integer arithmetic overflow issues, developers should utilize more extensive data types or conduct tests before calculating. This prevents values from exceeding lower data type limits.
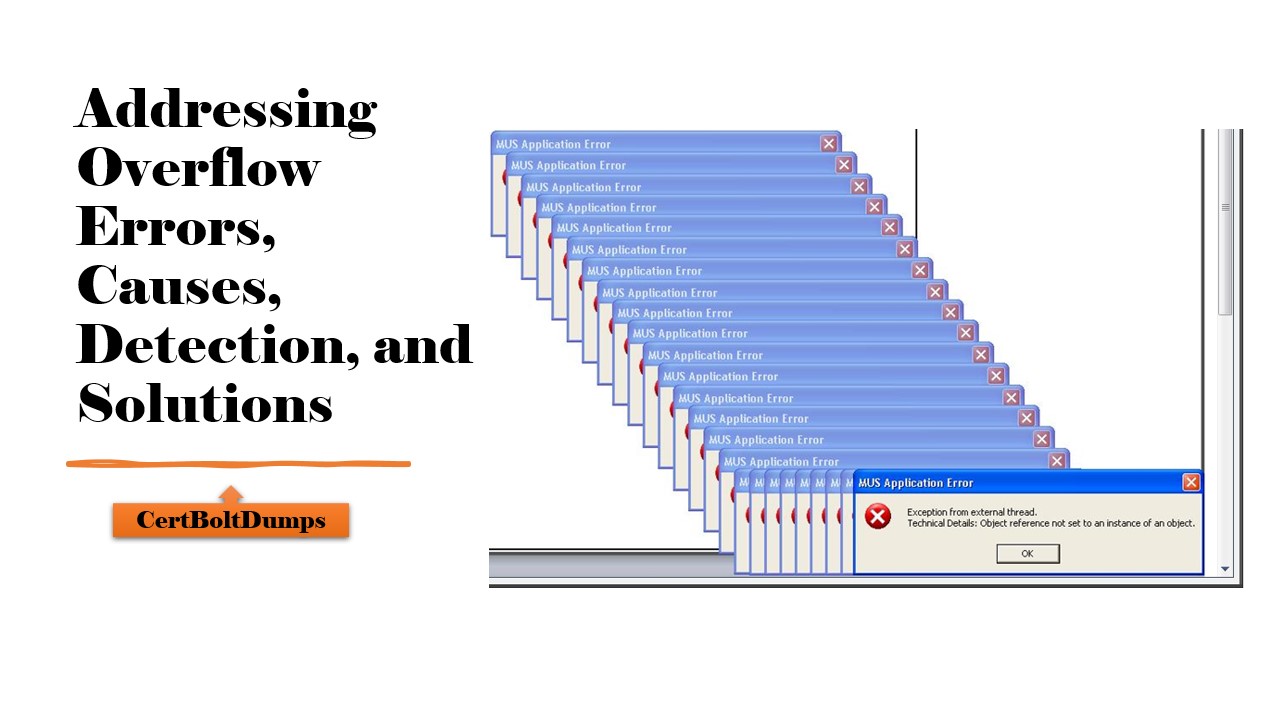
Input validation should always be used to verify that user-entered values fall within application-defined ranges. This reduces the chance of overflowing inputs generating errors.
Careful debugging and testing help detect and fix overflow issues during development rather than in production.
Developers may reduce overflow mistakes and build more dependable software by knowing these causes and implementing best practices, including variable selection, input validation, and thorough debugging.
Finding Overflow Errors
Detecting overflow issues is vital to fixing them. A value exceeding a data type's maximum limit causes these errors. Early overflow error detection is crucial for code integrity and operation.
To identify an overflow error, check if an arithmetic operation exceeds the data type's range of values. Compare the result to the lowest and maximum integer values for that type.
Building routines or libraries that manage overflows is another option. These tools usually detect overflows and act accordingly.
Some programming languages provide compiler flags or runtime checks that identify overflow faults during program execution. Enabling these features helps detect problems before they cause crashes or unusual behaviour.
Debugging methods like logging and error handling can also discover overflow faults. You may detect overflows before they create significant issues by monitoring variables and their values at important code places.
Remember, early discovery is crucial in fixing overflow issues. Be attentive throughout development by testing thoroughly and using tools intended to discover these vulnerabilities.
Fixing Overflow Errors
To fix an overflow error, find the source and apply solutions. Strategies to fix overflow errors:
- Validate input data before calculating or operating. Check and validate values to avoid exceeding thresholds.
- Use more extensive data types: For huge numbers or overflow-prone operations, use long integers or floating-point variables with more accuracy.
- Error handling: Use exception handling or try-catch blocks in your code. These properly manage possible overflows without software crashes.
- Range checking: Regularly verify variable values during computations and enforce bounds on outcomes that violate limitations.
- Optimization: Check your code's algorithms for efficiency and overflow issues. Reduce intermediate result overflow by optimizing algorithms by splitting complicated computations into more miniature stages.
Remember to be proactive while fixing overflow errors! Using these coding principles may reduce such mistakes and improve program performance.
Prevention of Overflow Errors
Preventing overflow faults is crucial. Here are some ways to avoid these pests.
First, select the correct data types for your variables. Use a smaller data type for variables that never exceed a range. You lessen overflow risk this way.
Verify external input before utilizing it in code. Check user input and API data for the anticipated range before calculating or operating on it.
Another successful method is to include error handling in your code. By predicting overflow possibilities and adding error checks and exception handling, you may gracefully manage them without crashing your software.
Break down complex calculations into smaller pieces or use intermediate variables when working with huge numbers or doing long computations. Reading is more accessible, and overflow issues are less likely to occur using this method.
Testing and debugging should occur throughout development. By checking your code for overflow faults early on, you may fix them before they become significant difficulties.
By proactively using these coding principles, you may reduce overflow problems and improve program performance.
Overflow Error Debugging Tips
- Examine the overflow error code first. Find computations or procedures that use huge numbers or variables with little data storage.
- Avoid overflow issues by selecting the suitable data types for your variables. Use a long or double instead of an int for a broader range of values.
- Check input values: User input and other data sources may create overflow issues. Validate inputs and handle edge situations to avoid surprises.
- Break complicated calculations: Break down complex formulae or equations in your code into smaller parts and verify intermediate results for overflow.
- Enable compiler warnings: Set your development environment to warn of potential overflow concerns during compilation. This helps uncover code issues before runtime.
- Log and debug: Use debug statements or log messages at important code places to track variable values and detect overflows.
- Wrap excess code with try-catch blocks to collect and handle errors gently instead of crashing the application.
Debugging is iterative, so keep going if you find the answer straight away! Be patient, systematic, and persistent while finding and fixing code overflow mistakes.
Examples and Solutions for Overflow Errors
In specific programming languages, overflow issues can cause unexpected outcomes or program failures. Let's examine overflow errors and their solutions.
First example:
An overflow error happens in basic arithmetic operations when the result exceeds the data type's maximum value. An overflow error occurs when we save a number more significant than an integer variable's total value. This may be fixed by using a more robust data type like long or double to store more significant numbers.
A second example:
Working with arrays or buffers is another typical case. Overflow errors occur when we write more data into a variety than its allotted size. To circumvent this problem, our code must check for length or size constraints before reporting data to these structures.
Example 3:
Loops and recursive routines can cause stack overflow issues. This happens when numerous function calls without termination conditions fill the call stack. To fix this, we should check our code logic and make sure loops and recursion have exit conditions.
These are some examples of overflow faults and their solutions. Developers must be aware of overflow risks while developing and make efforts to avoid them.
Handling Overflow Errors Best Practices
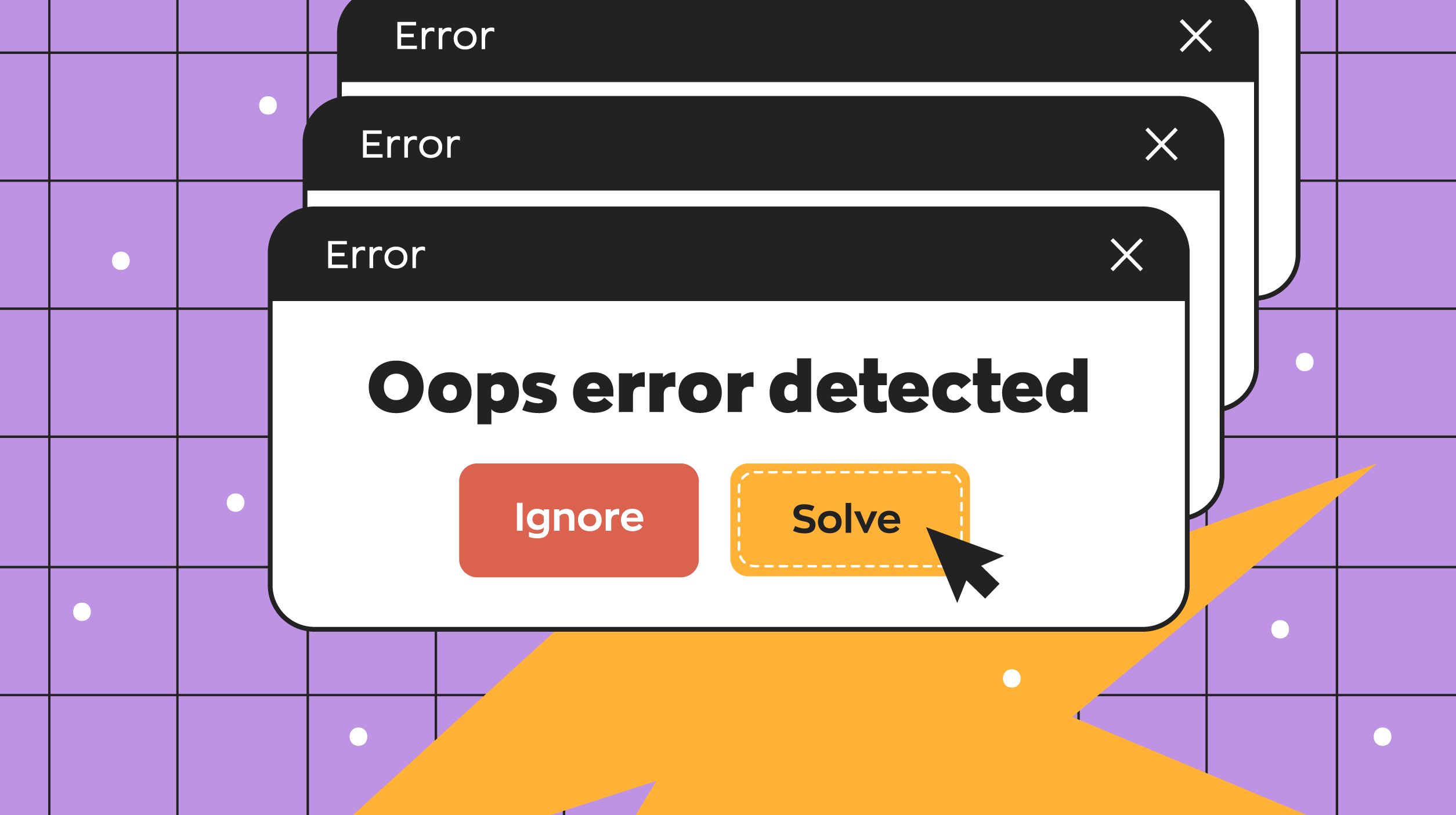
Some basic practices for addressing overflow errors might help your code run smoothly and avoid difficulties. Some effective methods:
- Use proper data types: Overflow issues are often caused by improper data storage. Select data types with enough range to handle expected values.
- Validate user input: Use input validation to prevent user values from exceeding the limit. This will prevent errors of overflow from causing surprises.
- review intermediate computations: Regularly review your code for intermediate calculations that might cause overflow errors. Monitoring these calculations lets you modify data type or add logic.
- Gracefully handle exceptions: Don't allow overflow errors to crash your program or trigger unwanted behaviour. Catch and address such issues using your programming language's exception handling.
- Before sending code into production: test rigorously to find and fix overflow issues. Create test cases to simulate overflows and verify that the code manages them.
By adopting these best practices, you may reduce the risk of software overflow issues causing disaster.
Students Benefit from Student-Centered Learning
Conclusion
Software stability and functioning depend on understanding and fixing overflow issues. Data type incompatibilities, mathematical computations, and memory constraints can cause these problems. Testing and debugging can help find these flaws early and improve them before they create significant concerns.
Overflow errors have numerous remedies depending on the situation. From changing data types to adding error handling, developers have several options. Developers may reduce overflow issues by following best practices and using input validation and memory allocation properly.
Overflow issues must be debugged by evaluating code logic, checking computations step by step, and applying anomaly detection tools. Unexpected program behaviour or improper output values may signal an overflow issue, so pay carefully.
We used integer division, array indexing, and floating-point arithmetic to demonstrate overflow issues and offer remedies. These examples illustrate the significance of careful development and testing to minimize overflow issues.
Understanding overflow issues, recognizing them early, fixing them, and preventing them can help ensure robust software development. Stay diligent, pay attention to detail, and follow best practices to control bugs and create high-quality, error-free apps!
Comments (0)